Assets & Fonts
- How to include assets in your app :
-
Create an
assets/images folder -
This should be located in the root of your project, in the same folder as your
pubspec.yaml file. -
You don't have to call it
assets orimages . You don't even need to make images a subfolder. Whatever name you use, though, is what you will regester in thepubspec.yaml file. - Add your image to the new folder
- Register the assets folder in
pubspec.yaml -
Open the pubspec.yaml file that is in the root of your project.
-
Add an
assets subsection to theflutter section like this: -
If you have multiple images that you want to include then you can leave off the file name and just use the directory name (include the final
/ ): - Use the image in code
- Restart your app
- Use a custom font :
- Import the font files.
- Declare the font in the pubspec.
- Set a font as the default.
- Use a font in a specific widget.
(Images for example)
You can just copy your image into assets/images. The relative path of heart.jpg, for example, would be
flutter:
assets:
- assets/images/heart.jpg
flutter:
assets:
- assets/images/
Get the asset in an Image widget with
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Asset Image Test',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: HomeWidget());
}
}
class HomeWidget extends StatelessWidget {
const HomeWidget({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Asset Image"),
),
body: Image.asset("assets/images/heart.jpg"));
}
}
When making changes to pubspec.yaml, you may often need to completely stop the app and restart it
again, especially when adding assets. Otherwise you may get a crash.
Running the app now you should have this:
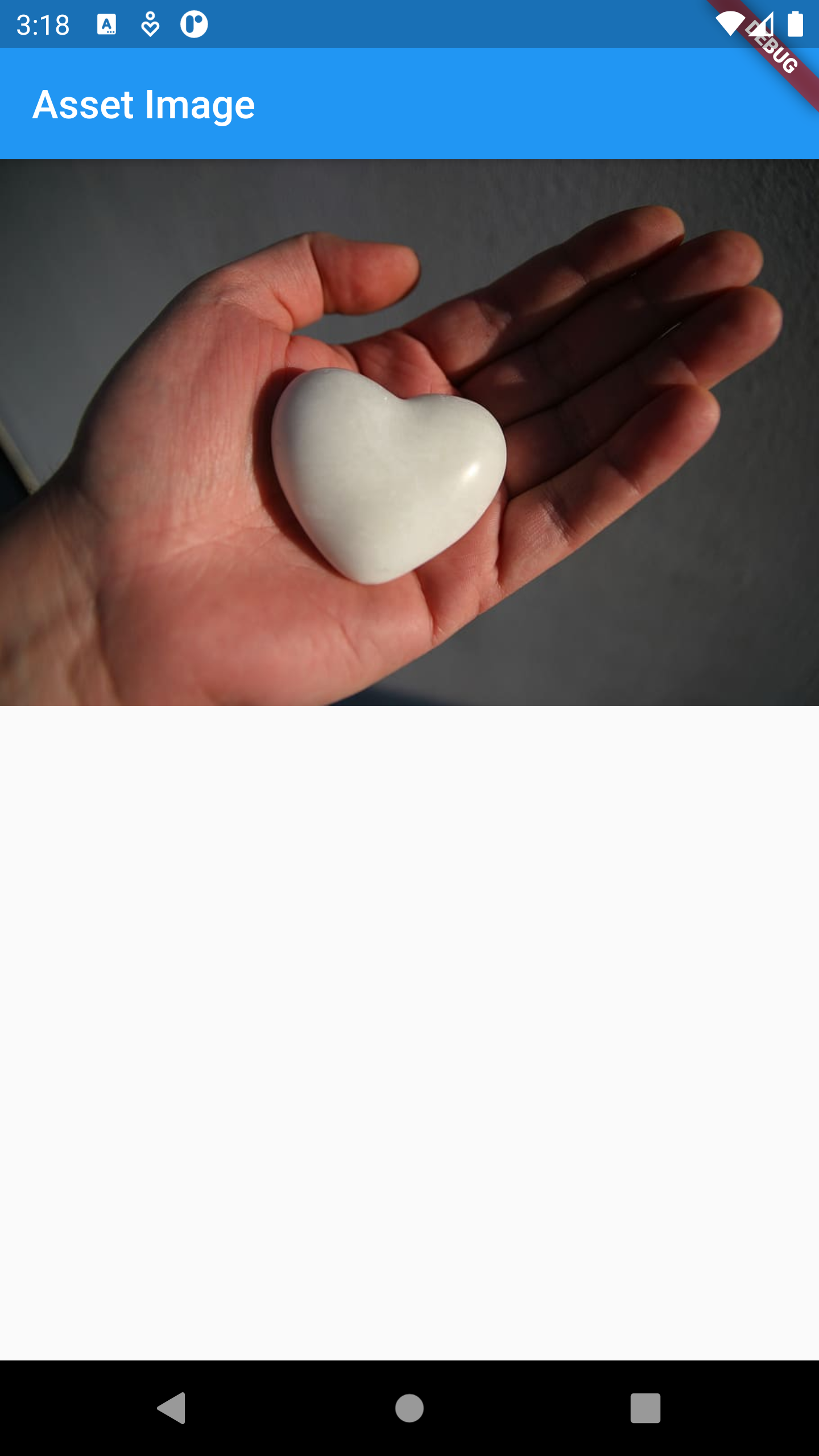
To work with a font, import the font files into the project. It’s common practice to put font files in a
Supported font formats : .ttf & .otf
Visit Google Fonts and choose the font that you like.
Once you’ve identified a font, tell Flutter where to find it. You can do this by including a font definition in the pubspec.yaml file.
flutter:
fonts:
- family: Audiowide
fonts:
- asset: assets/fonts/Audiowide-Regular.ttf
The
The
You have two options for how to apply fonts to text: as the default font or only within specific widgets.
To use a font as the default, set the fontFamily property as part of the app’s theme. The value provided to fontFamily must match the family name declared in the pubspec.yaml.
MaterialApp(
title: 'Custom Font',
theme: ThemeData(fontFamily: 'Audiowide'),
home: HomeWidget()
);
If you want to apply the font to a specific widget, such as a Text widget, provide a TextStyle to the widget.
Text(
"Lorem Ipsum is simply dummy text of the printing and typesetting industry.",
style: TextStyle(fontFamily: "Audiowide"),
)
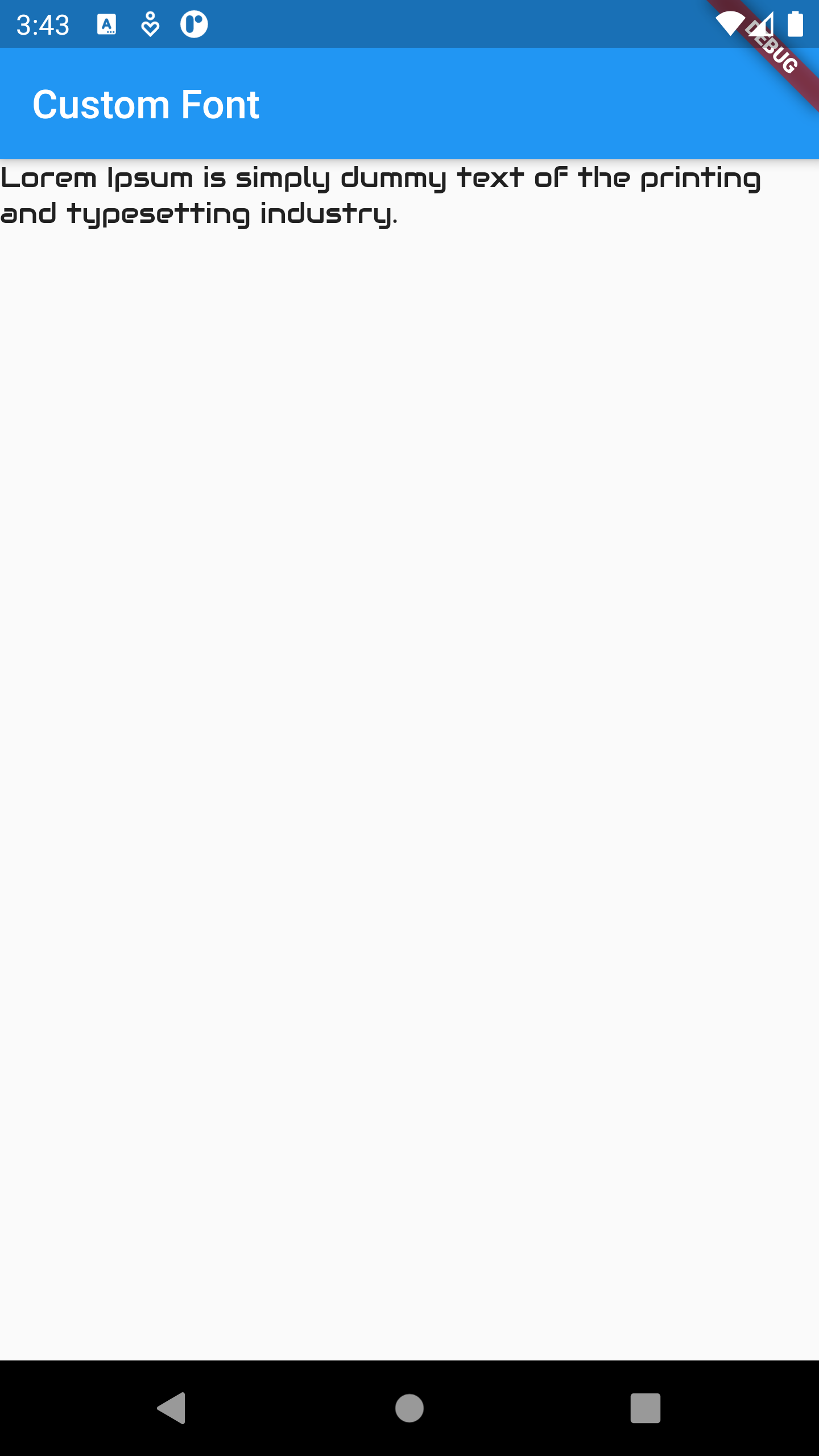