App7 : Short Vacation - Learning New Widgets
Table Of Content :
- OutlinedButton
- child : Widget
- onPressed :
- style : ButtonStyle?
- CupertinoDatePicker
- backgroundColor : Color?
- initialDateTime : DateTime
- maximumDate : DateTime?
- minimumDate : DateTime?
- mode : CupertinoDatePickerMode
- onDateTimeChanged : Widget?
A Material Design "Outlined Button"; essentially a TextButton with an outlined border.
Outlined buttons are medium-emphasis buttons. They contain actions that are important, but they aren’t the
primary action in an app.
Some properties of CupertinoButton Class:
Typically the button's label.
Called when the button is tapped or otherwise activated.
Customizes this button's appearance.
Example :
OutlinedButton(
child: Text("Click Me"),
onPressed: () {
print("clicked");
},
style: TextButton.styleFrom(backgroundColor: Colors.white38),
),
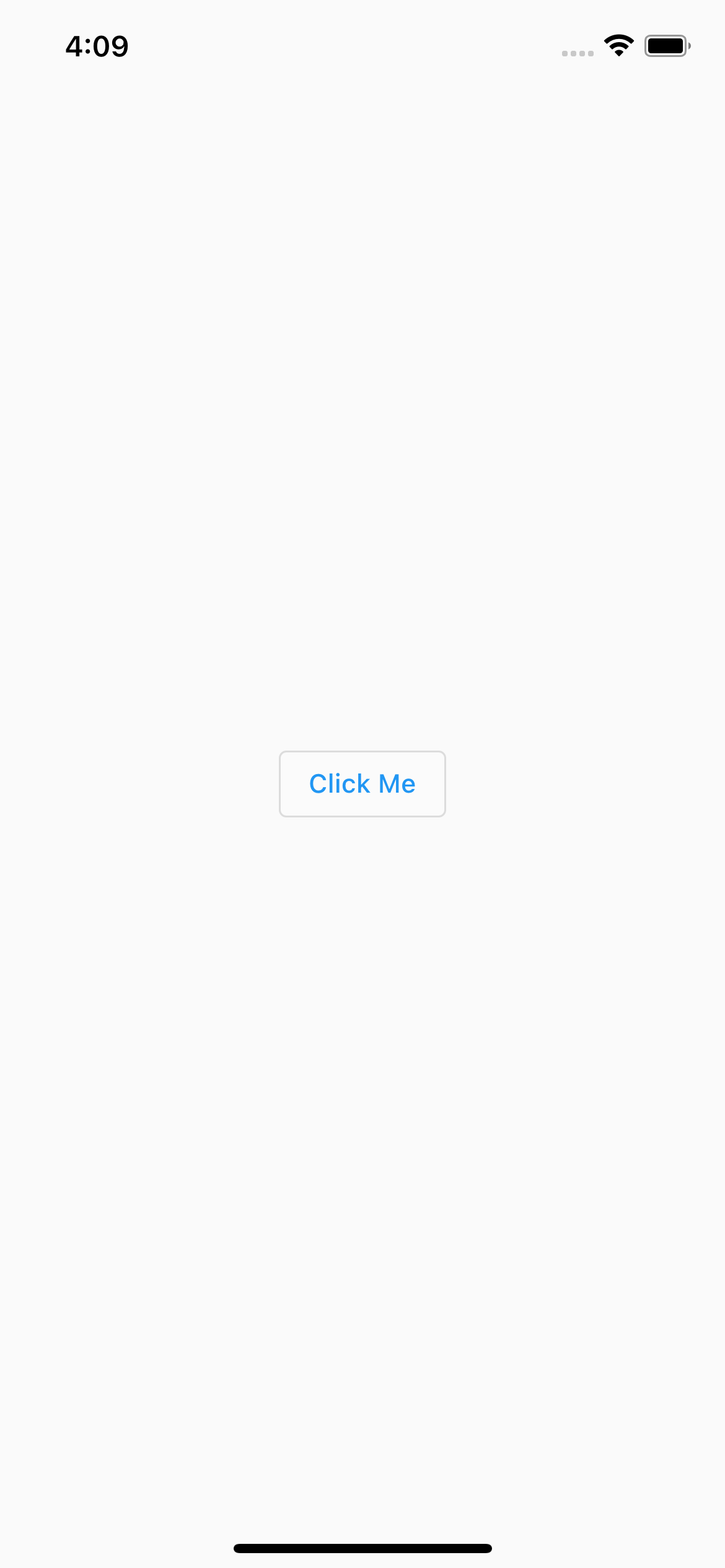
A date picker widget in iOS style.
There are several modes of the date picker listed in CupertinoDatePickerMode.
The class will display its children as consecutive columns. Its children order is based on
internationalization, or the dateOrder property if specified.
Some properties of CupertinoDatePicker Class:
Background color of date picker.
The initial date and/or time of the picker. Defaults to the present date and time and must not be null. The present must conform to the intervals set in minimumDate, maximumDate, minimumYear, and maximumYear.
The maximum selectable date that the picker can settle on.
The minimum selectable date that the picker can settle on.
The mode of the date picker as one of CupertinoDatePickerMode. Defaults to CupertinoDatePickerMode.dateAndTime. Cannot be null and value cannot change after initial build.
Callback called when the selected date and/or time changes. If the new selected DateTime is not valid, or is not in the minimumDate through maximumDate range, this callback will not be called.
Example :
DateTime date =DateTime.now();
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: CupertinoDatePicker(
backgroundColor: Colors.lime,
initialDateTime: DateTime.now(),
maximumDate: DateTime.now().add(Duration(days: 365)),
minimumDate: DateTime.now().subtract(Duration(days: 30)),
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (newDate){
date=newDate;
}
,
)
),
);
}
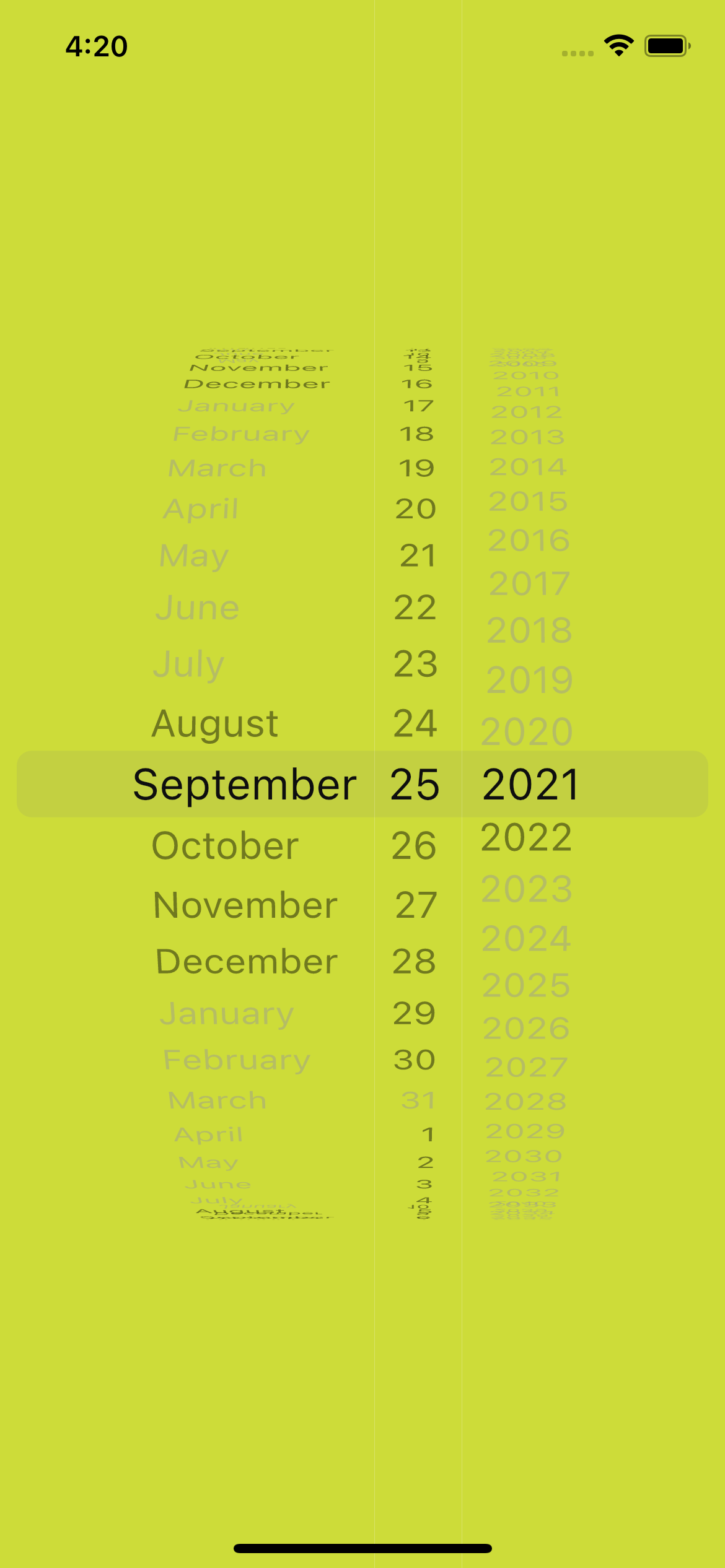