App8 : Movie Time - Learning New Widgets
- GridView
- Table
- border : TableBorder?
- children : List<TableRow>
- columnWidths : Map<int, TableColumnWidth>?
- defaultColumnWidth : TableColumnWidth
- defaultVerticalAlignment : TableCellVerticalAlignment
A scrollable, 2D array of widgets.
The main axis direction of a grid is the direction in which it scrolls (the scrollDirection).
The most commonly used grid layouts are GridView.count, which creates a layout
with a fixed number of tiles in the cross axis, and GridView.extent, which creates
a layout with tiles that have a maximum cross-axis extent.
To create a grid with a large (or infinite) number of children, use the GridView.builder. .
Example :
class MainPage extends StatelessWidget {
MainPage({Key? key}) : super(key: key);
final colors = [
Colors.red,
Colors.pink,
Colors.purple,
Colors.deepPurple,
Colors.indigo,
Colors.blue,
Colors.lightBlue,
Colors.cyan,
Colors.teal,
Colors.green,
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter GridView'),
),
body: GridView.count(
crossAxisCount: 3,
childAspectRatio: 1,
children: List.generate(colors.length, (index) {
return Container(
color: colors[index],
margin: EdgeInsets.all(20),
child: Center(child: Text(index.toString())),
);
}),
),
);
}
}
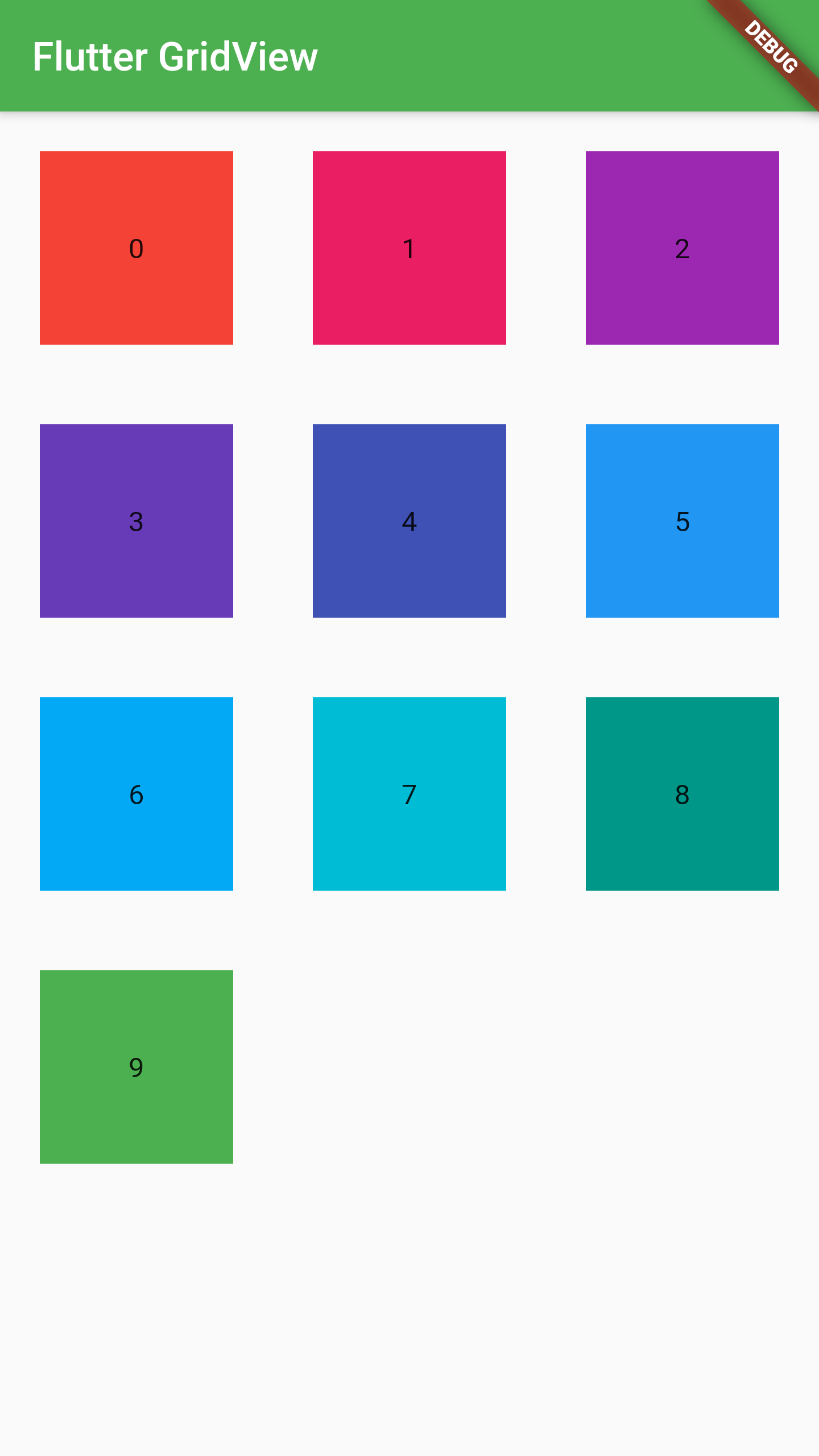
A widget that uses the table layout algorithm for its children.
Some properties of Table Class:
The style to use when painting the boundary and interior divisions of the table.
The rows of the table.
How the horizontal extents of the columns of this table should be determined.
How to determine with widths of columns that don't have an explicit sizing algorithm.
How cells that do not explicitly specify a vertical alignment are aligned vertically.
Example :
Table(
border: TableBorder.all(color: Colors.deepOrange, width: 2),
columnWidths: const {
0: FractionColumnWidth(0.6),
1: FractionColumnWidth(0.4)
},
defaultVerticalAlignment: TableCellVerticalAlignment.middle,
children: const [
TableRow(children: [Text("Netherlands"), Text("Amsterdam")]),
TableRow(children: [Text("Greece"), Text("Athens")]),
TableRow(children: [Text("Germany"), Text("Berlin")]),
TableRow(children: [Text("Denmark"), Text("Copenhagen ")]),
TableRow(children: [Text("Norway"), Text("Oslo")]),
],
),
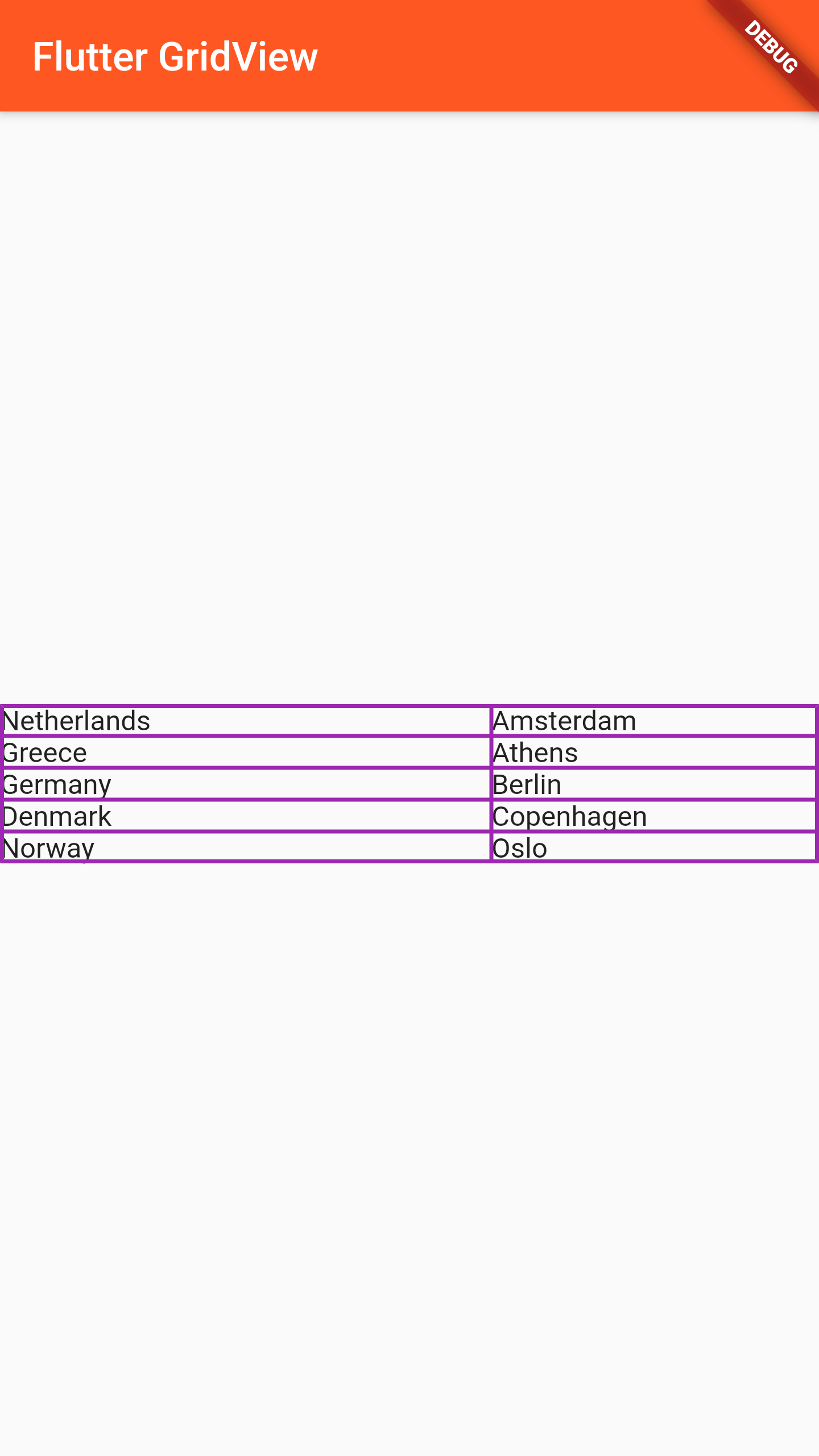