Device Orientation
- Lock rotation in the entire app
- Detect Orientation Change in Layout
Quite often an application is developed only for single orientation, for example — portrait. But by default
an application changes orientation when you rotate a device.
To change this behaviour we need to explicitly define supported orientation.
void main() {
WidgetsFlutterBinding.ensureInitialized();
SystemChrome.setPreferredOrientations(
//1. Portrait mode:
// [DeviceOrientation.portraitUp, DeviceOrientation.portraitDown]
//2. Landscape mode:
[DeviceOrientation.landscapeLeft, DeviceOrientation.landscapeRight])
.then((_) => runApp(const MyApp()));
}
Note:
There are a small problem which is the application changes orientation after the launch :
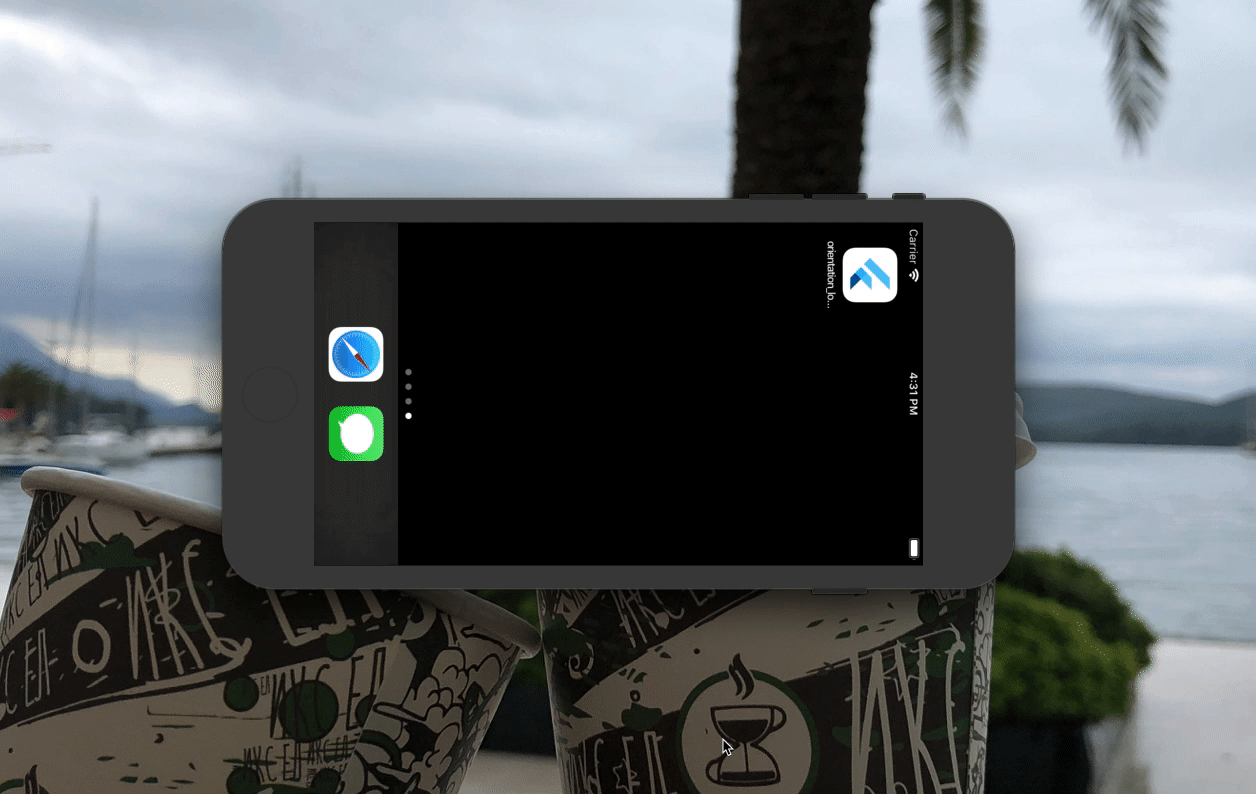
To solve this problem check this Article on Medium.
Now we can use MediaQuery to check orientation:
Scaffold(
appBar: AppBar(title: const Text('Device Orientation')),
body: MediaQuery.of(context).orientation == Orientation.portrait
? Container(
alignment: Alignment.center,
margin: const EdgeInsets.all(20),
height: 200,
color: Colors.blueAccent,
child: const Text(
'Portrait Screen',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
)
: Container(
alignment: Alignment.center,
margin: const EdgeInsets.all(20),
height: 200,
color: Colors.deepOrange,
child: const Text(
'Landscape Screen',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
))
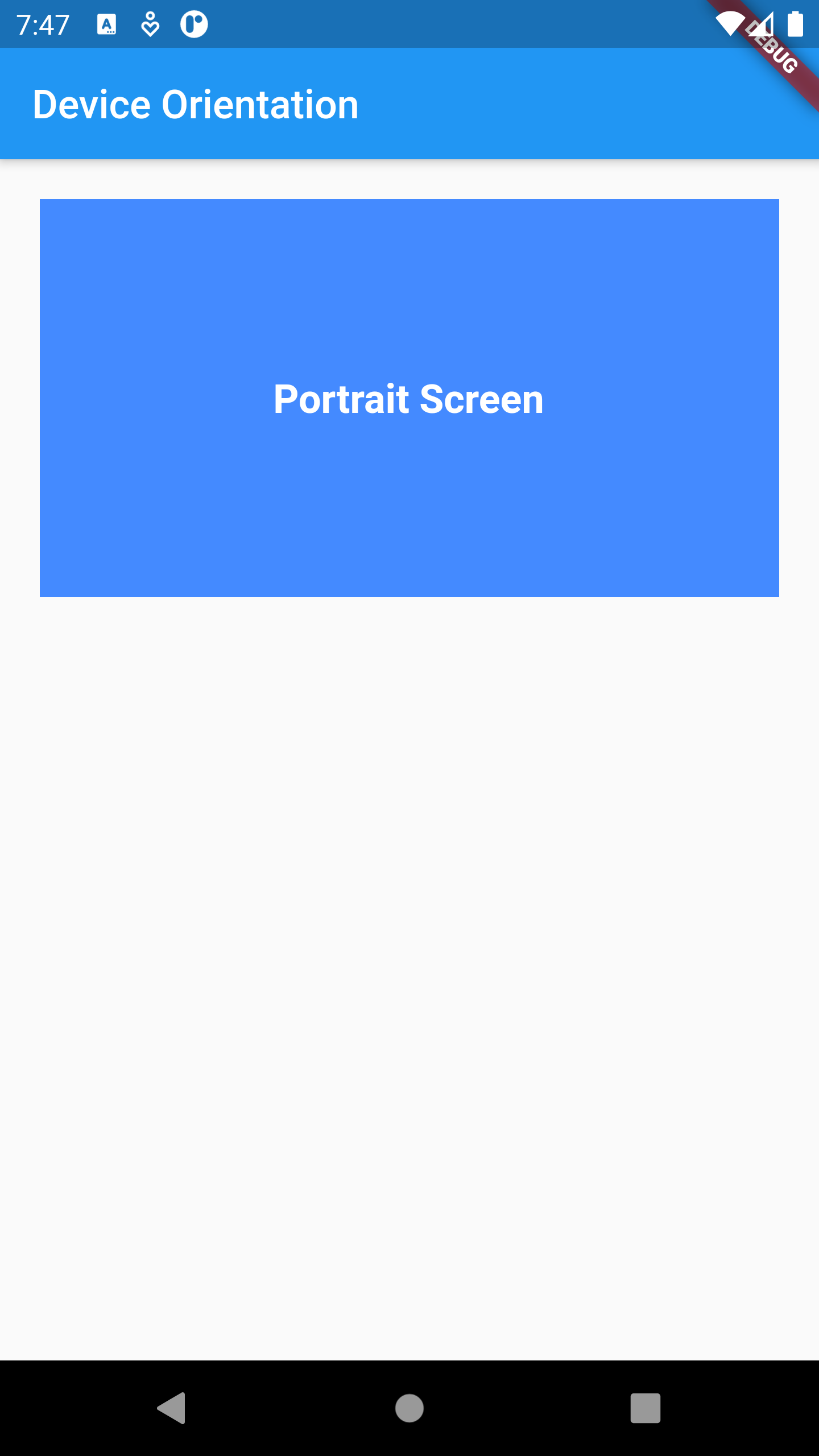
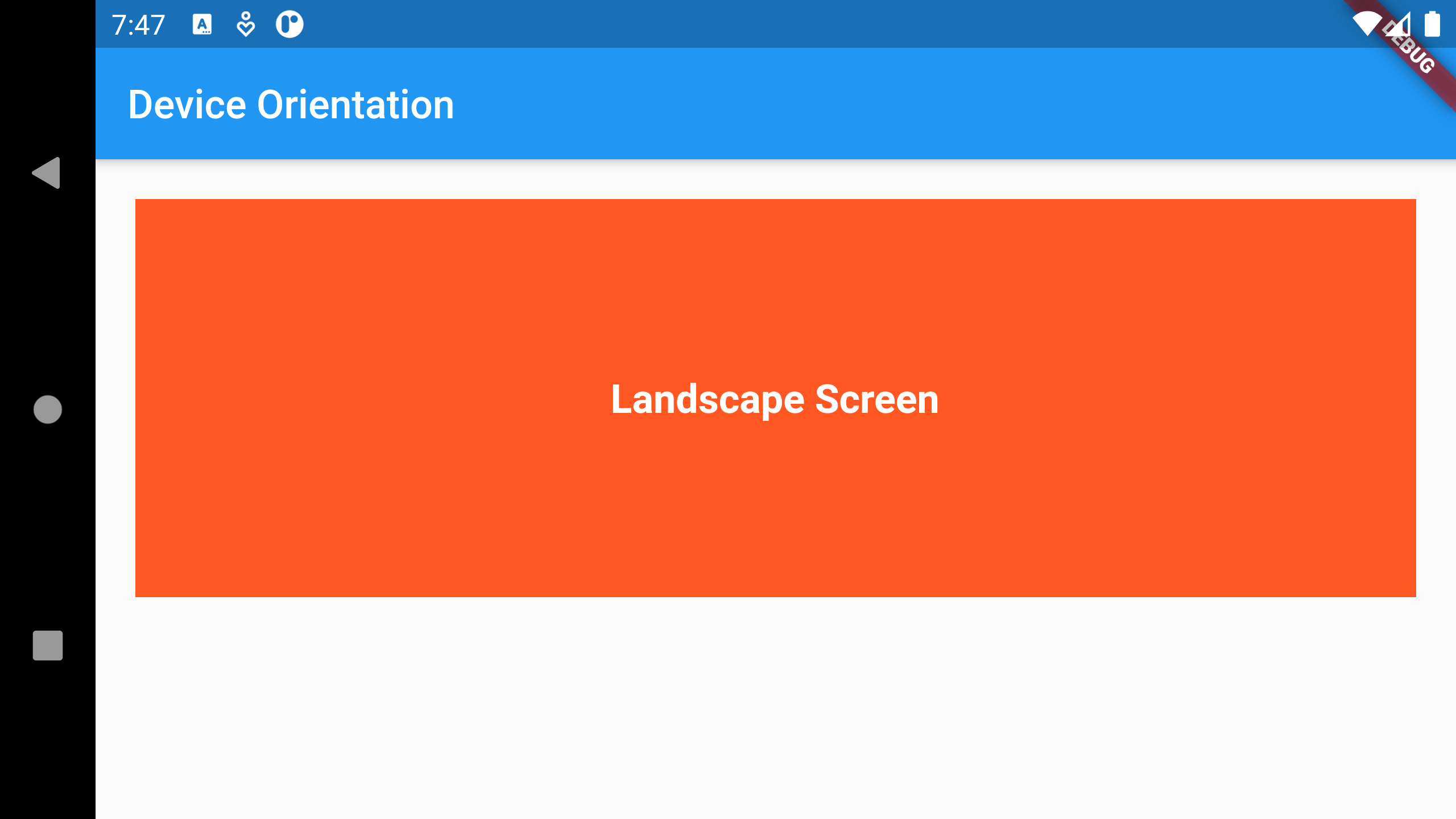