The Weather Model Class
- Getting the API key
-
Create an account in OpenWeather and go to the API page.
-
Click subscribe for the Daily Forecast 16 days and get the API key.
-
Save the api key as a static const in a separated file.
- Creating The Weather Model Class
-
Visit this link 5 day weather forecast
-
Remplace this field with your api key and test this link in your browser
-
We will use a very practical tool that transform the json model to a dart model.
visit JSON to Dart.
Paste the response and type OpenWeather in class name field.
-
Copy the result in a new file.
-
You will see some errors caused by a class name called List which is a keword in Dart, so we need to change it to Liste .
Also change the double type to num.
You can copy the result from here :
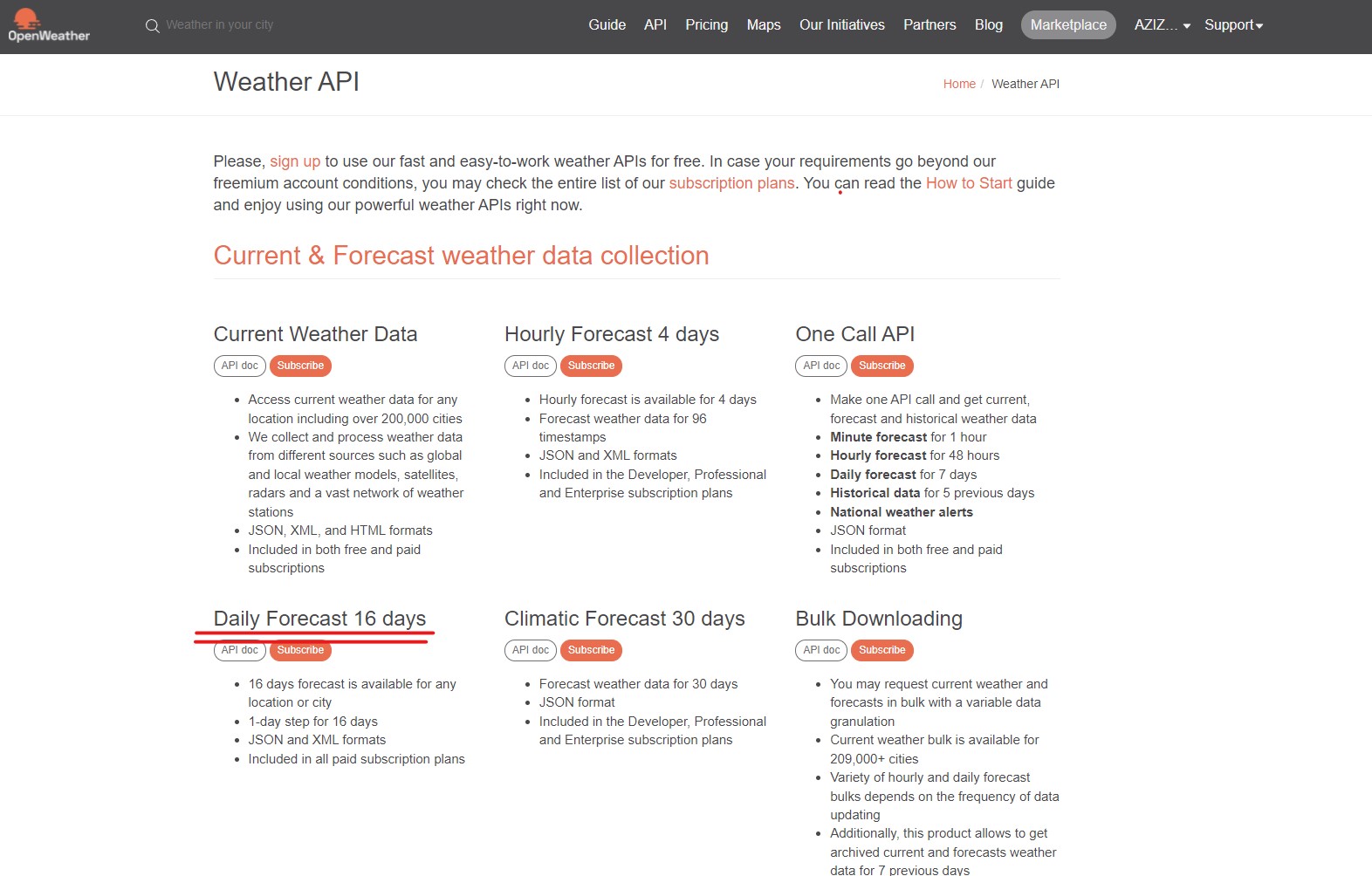
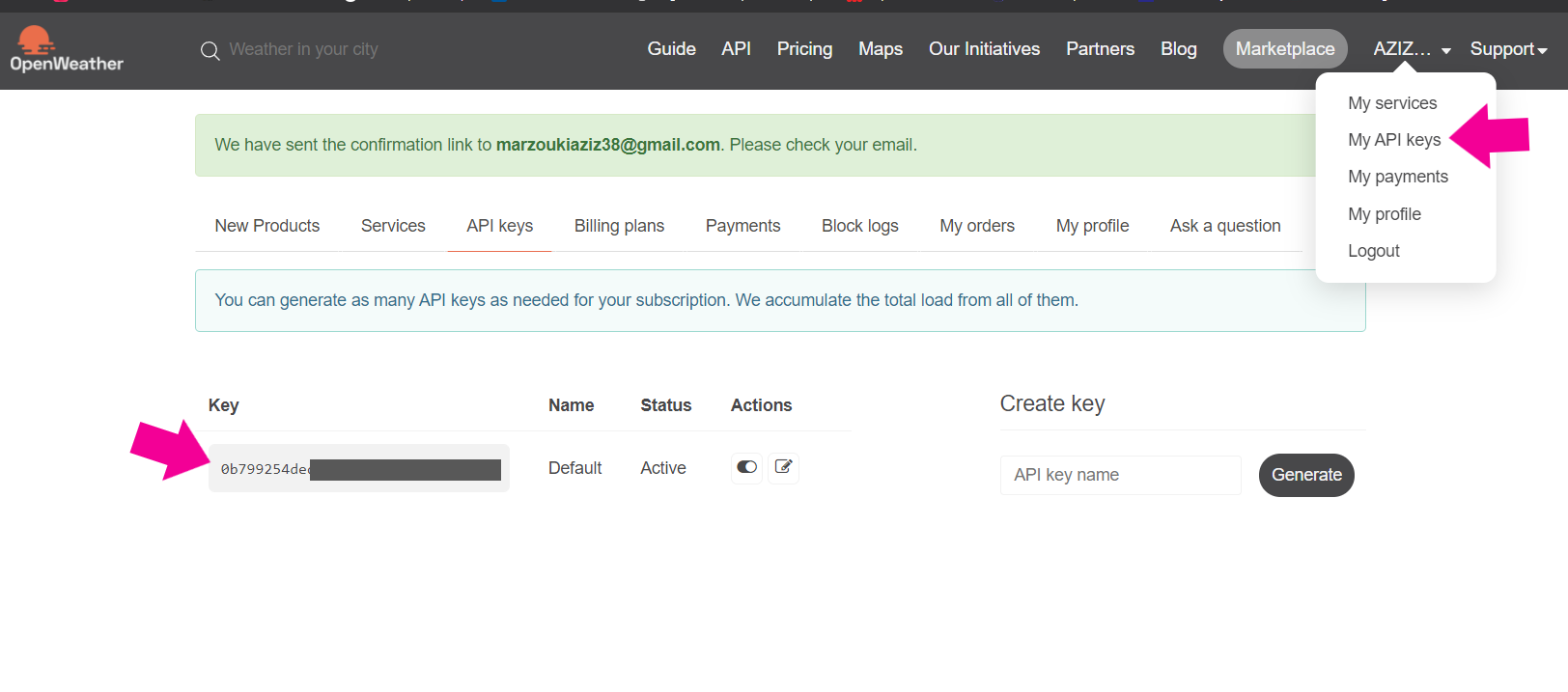
class ApiKey {
static const String OPEN_WEATHER_API = " < Your-API-Key > ";
}
api.openweathermap.org/data/2.5/forecast?q=london,DE&appid={API key}
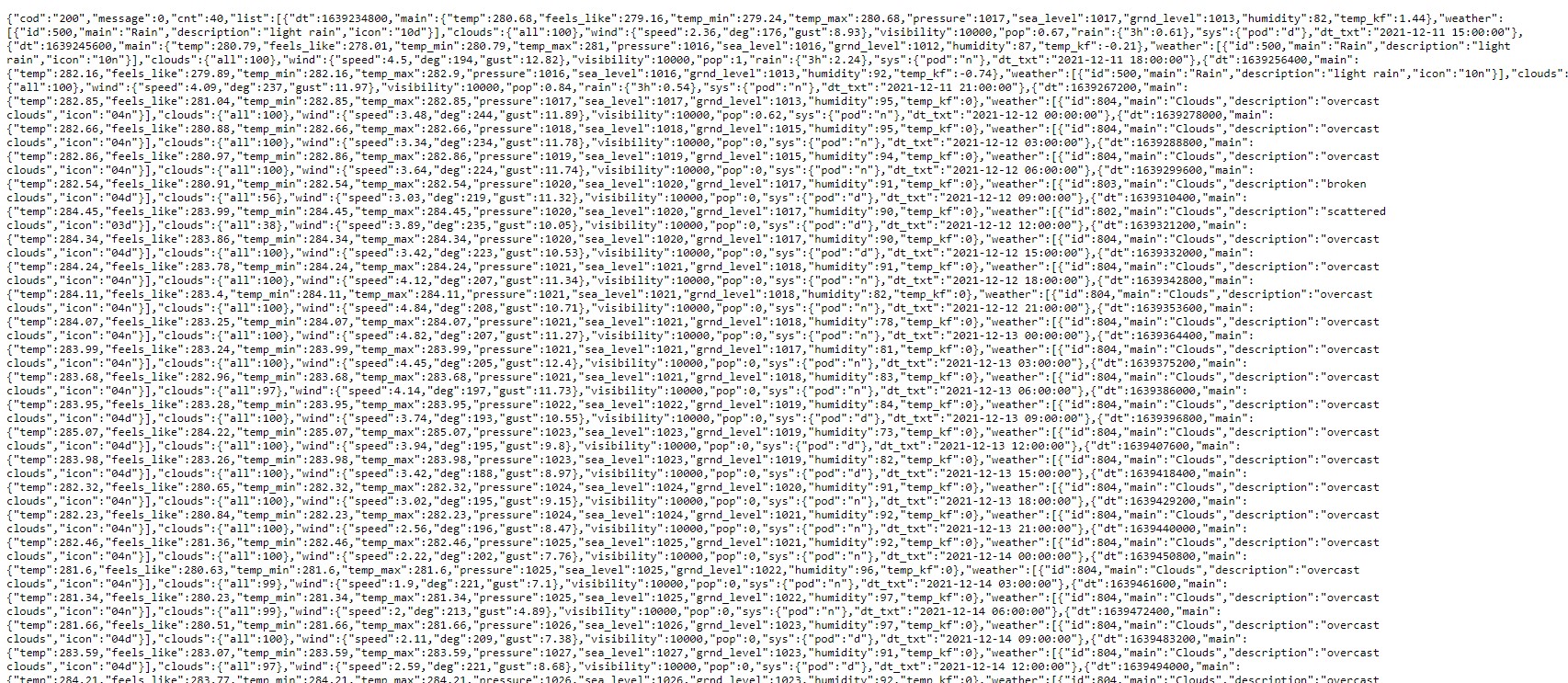
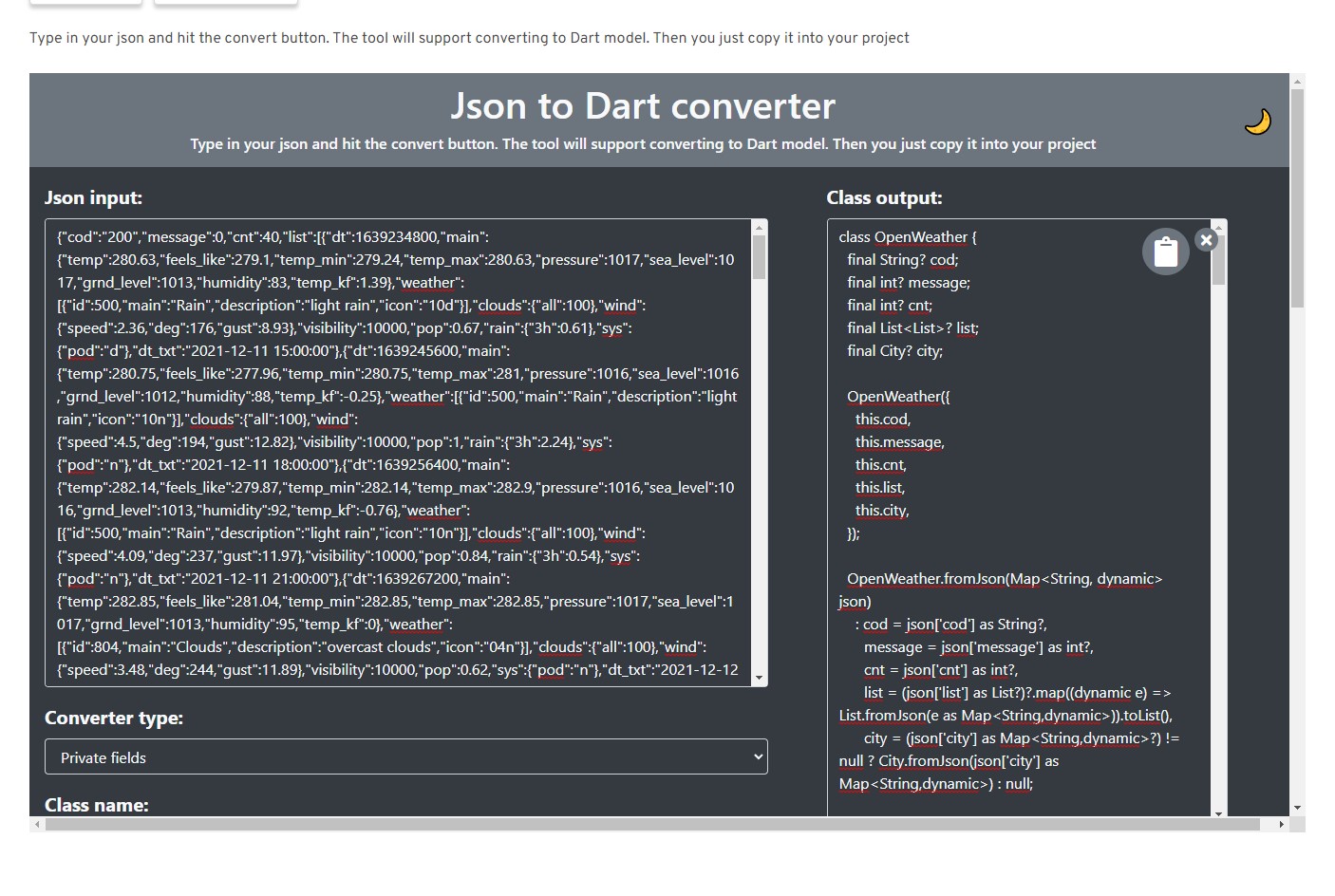
class OpenWeather {
final String? cod;
final int? message;
final int? cnt;
final List<Liste>? list;
final City? city;
OpenWeather({
this.cod,
this.message,
this.cnt,
this.list,
this.city,
});
OpenWeather.fromJson(Map<String, dynamic> json)
: cod = json['cod'] as String?,
message = json['message'] as int?,
cnt = json['cnt'] as int?,
list = (json['list'] as List?)
?.map((dynamic e) => Liste.fromJson(e as Map<String, dynamic>))
.toList(),
city = (json['city'] as Map<String, dynamic>?) != null
? City.fromJson(json['city'] as Map<String, dynamic>)
: null;
Map<String, dynamic> toJson() => {
'cod': cod,
'message': message,
'cnt': cnt,
'list': list?.map((e) => e.toJson()).toList(),
'city': city?.toJson()
};
}
class Liste {
final int? dt;
final Main? main;
final List<Weather>? weather;
final Clouds? clouds;
final Wind? wind;
final int? visibility;
final double? pop;
final Rain? rain;
final Sys? sys;
final String? dtTxt;
Liste({
this.dt,
this.main,
this.weather,
this.clouds,
this.wind,
this.visibility,
this.pop,
this.rain,
this.sys,
this.dtTxt,
});
Liste.fromJson(Map<String, dynamic> json)
: dt = json['dt'] as int?,
main = (json['main'] as Map<String, dynamic>?) != null
? Main.fromJson(json['main'] as Map<String, dynamic>)
: null,
weather = (json['weather'] as List?)
?.map((dynamic e) => Weather.fromJson(e as Map<String, dynamic>))
.toList(),
clouds = (json['clouds'] as Map<String, dynamic>?) != null
? Clouds.fromJson(json['clouds'] as Map<String, dynamic>)
: null,
wind = (json['wind'] as Map<String, dynamic>?) != null
? Wind.fromJson(json['wind'] as Map<String, dynamic>)
: null,
visibility = json['visibility'] as int?,
pop = json['pop'] as double?,
rain = (json['rain'] as Map<String, dynamic>?) != null
? Rain.fromJson(json['rain'] as Map<String, dynamic>)
: null,
sys = (json['sys'] as Map<String, dynamic>?) != null
? Sys.fromJson(json['sys'] as Map<String, dynamic>)
: null,
dtTxt = json['dt_txt'] as String?;
Map<String, dynamic> toJson() => {
'dt': dt,
'main': main?.toJson(),
'weather': weather?.map((e) => e.toJson()).toList(),
'clouds': clouds?.toJson(),
'wind': wind?.toJson(),
'visibility': visibility,
'pop': pop,
'rain': rain?.toJson(),
'sys': sys?.toJson(),
'dt_txt': dtTxt
};
}
class Main {
final double? temp;
final double? feelsLike;
final double? tempMin;
final double? tempMax;
final int? pressure;
final int? seaLevel;
final int? grndLevel;
final int? humidity;
final double? tempKf;
Main({
this.temp,
this.feelsLike,
this.tempMin,
this.tempMax,
this.pressure,
this.seaLevel,
this.grndLevel,
this.humidity,
this.tempKf,
});
Main.fromJson(Map<String, dynamic> json)
: temp = json['temp'] as double?,
feelsLike = json['feels_like'] as double?,
tempMin = json['temp_min'] as double?,
tempMax = json['temp_max'] as double?,
pressure = json['pressure'] as int?,
seaLevel = json['sea_level'] as int?,
grndLevel = json['grnd_level'] as int?,
humidity = json['humidity'] as int?,
tempKf = json['temp_kf'] as double?;
Map<String, dynamic> toJson() => {
'temp': temp,
'feels_like': feelsLike,
'temp_min': tempMin,
'temp_max': tempMax,
'pressure': pressure,
'sea_level': seaLevel,
'grnd_level': grndLevel,
'humidity': humidity,
'temp_kf': tempKf
};
}
class Weather {
final int? id;
final String? main;
final String? description;
final String? icon;
Weather({
this.id,
this.main,
this.description,
this.icon,
});
Weather.fromJson(Map<String, dynamic> json)
: id = json['id'] as int?,
main = json['main'] as String?,
description = json['description'] as String?,
icon = json['icon'] as String?;
Map<String, dynamic> toJson() =>
{'id': id, 'main': main, 'description': description, 'icon': icon};
}
class Clouds {
final int? all;
Clouds({
this.all,
});
Clouds.fromJson(Map<String, dynamic> json) : all = json['all'] as int?;
Map<String, dynamic> toJson() => {'all': all};
}
class Wind {
final double? speed;
final int? deg;
final double? gust;
Wind({
this.speed,
this.deg,
this.gust,
});
Wind.fromJson(Map<String, dynamic> json)
: speed = json['speed'] as double?,
deg = json['deg'] as int?,
gust = json['gust'] as double?;
Map<String, dynamic> toJson() => {'speed': speed, 'deg': deg, 'gust': gust};
}
class Rain {
final double? h;
Rain({
this.h,
});
Rain.fromJson(Map<String, dynamic> json) : h = json['3h'] as double?;
Map<String, dynamic> toJson() => {'3h': h};
}
class Sys {
final String? pod;
Sys({
this.pod,
});
Sys.fromJson(Map<String, dynamic> json) : pod = json['pod'] as String?;
Map<String, dynamic> toJson() => {'pod': pod};
}
class City {
final int? id;
final String? name;
final Coord? coord;
final String? country;
final int? population;
final int? timezone;
final int? sunrise;
final int? sunset;
City({
this.id,
this.name,
this.coord,
this.country,
this.population,
this.timezone,
this.sunrise,
this.sunset,
});
City.fromJson(Map<String, dynamic> json)
: id = json['id'] as int?,
name = json['name'] as String?,
coord = (json['coord'] as Map<String, dynamic>?) != null
? Coord.fromJson(json['coord'] as Map<String, dynamic>)
: null,
country = json['country'] as String?,
population = json['population'] as int?,
timezone = json['timezone'] as int?,
sunrise = json['sunrise'] as int?,
sunset = json['sunset'] as int?;
Map<String, dynamic> toJson() => {
'id': id,
'name': name,
'coord': coord?.toJson(),
'country': country,
'population': population,
'timezone': timezone,
'sunrise': sunrise,
'sunset': sunset
};
}
class Coord {
final double? lat;
final double? lon;
Coord({
this.lat,
this.lon,
});
Coord.fromJson(Map<String, dynamic> json)
: lat = json['lat'] as double?,
lon = json['lon'] as double?;
Map<String, dynamic> toJson() => {'lat': lat, 'lon': lon};
}
Fields in API response
· cod Internal parameter
· message Internal parameter
· cntA number of timestamps returned in the API response
· list
o list.dt Time of data forecasted, unix, UTC
o list.main
§ list.main.temp Temperature. Unit Default: Kelvin, Metric: Celsius, Imperial: Fahrenheit.
§ list.main.feels_like This temperature parameter accounts for the human perception of weather. Unit Default: Kelvin, Metric: Celsius, Imperial: Fahrenheit.
§ list.main.temp_min Minimum temperature at the moment of calculation. This is minimal forecasted temperature (within large megalopolises and urban areas), use this parameter optionally. Unit Default: Kelvin, Metric: Celsius, Imperial: Fahrenheit.
§ list.main.temp_max Maximum temperature at the moment of calculation. This is maximal forecasted temperature (within large megalopolises and urban areas), use this parameter optionally. Unit Default: Kelvin, Metric: Celsius, Imperial: Fahrenheit.
§ list.main.pressure Atmospheric pressure on the sea level by default, hPa
§ list.main.sea_level Atmospheric pressure on the sea level, hPa
§ list.main.grnd_level Atmospheric pressure on the ground level, hPa
§ list.main.humidity Humidity, %
§ list.main.temp_kf Internal parameter
o list.weather
§ list.weather.id Weather condition id
§ list.weather.main Group of weather parameters (Rain, Snow, Extreme etc.)
§ list.weather.description Weather condition within the group. You can get the output in your language. Learn more.
§ list.weather.icon Weather icon id
o list.clouds
§ list.clouds.all Cloudiness, %
o list.wind
§ list.wind.speed Wind speed. Unit Default: meter/sec, Metric: meter/sec, Imperial: miles/hour.
§ list.wind.deg Wind direction, degrees (meteorological)
§ list.wind.gust Wind gust. Unit Default: meter/sec, Metric: meter/sec, Imperial: miles/hour
o list.visibility Average visibility, metres
o list.pop Probability of precipitation
o list.rain
§ list.rain.3h Rain volume for last 3 hours, mm
o list.snow
§ list.snow.3h Snow volume for last 3 hours
o list.sys
§ list.sys.pod Part of the day (n - night, d - day)
o list.dt_txt Time of data forecasted, ISO, UTC
· city
o city.id City ID
o city.name City name
o city.coord
§ city.coord.lat City geo location, latitude
§ city.coord.lon City geo location, longitude
o city.country Country code (GB, JP etc.)
o city.timezone Shift in seconds from UTC