Adding Shared Preferences To App8
We will store the list of favorite movies by saving their ids using shared preferences.
- Installing
- Import the package in /models/movie.dart
- Create a key and list of ids
- Adding Movie To Favorites by updating the addMovieToFavorite method
- Getting The List Of Favorite Movies
- Test the app
- Code Source
Run this command:
flutter pub add shared_preferences
This will add a line like this to your package's pubspec.yaml (and run an implicit flutter pub get):
dependencies:
shared_preferences: ^2.0.9
Or visit shared_preferences - pub.dev
Now run pub get from the command line:
flutter pub get
You’re now ready to store data.
import 'package:shared_preferences/shared_preferences.dart';
All preferences need to use a unique key or they’ll be overwritten. Here, you’re simply defining a constant for the preference key.
static const String favMoviesKey = 'favMovies';
static List<String> favMoviesIds = [];
Here, you use the async keyword to indicate that this method will run
asynchronously. It also:
1. Uses the await keyword to wait for an instance of SharedPreferences.
2. Adding the id of the movie to favMoviesIds
3. Saves the new list using the favMoviesKey.
static void addMovieToFavorite(Movie m) async {
//1
final prefs = await SharedPreferences.getInstance();
//2
favMoviesIds.add(m.id.toString());
//3
prefs.setStringList(favMoviesKey, favMoviesIds);
_favoriteMovies.add(m);
}
static void getFavMoviesFromSharedPreferences() async {
//1
final prefs = await SharedPreferences.getInstance();
//2
if (prefs.containsKey(favMoviesKey)) {
//3
favMoviesIds = prefs.getStringList(favMoviesKey)!;
//4
for (String id in favMoviesIds) {
_favoriteMovies
.add(movies.firstWhere((movie) => movie.id.toString() == id));
}
}
}
This method is also asynchronous. Here, you:
1. Use the await keyword to wait for an instance of SharedPreferences.
2. Check if a preference for the list of favorite movies already exists.
3. Get the list.
4. Use the id to load the movie object in the _favoriteMovies list.
Finally, call it before runApp(const MyApp()) to load the list when the app starts:
import './models/movie.dart';
void main() {
//locking orientaiton
WidgetsFlutterBinding.ensureInitialized();
SystemChrome.setPreferredOrientations(
[DeviceOrientation.portraitDown, DeviceOrientation.portraitUp]).then((_) {
//add it here
Movie.getFavMoviesFromSharedPreferences();
//
runApp(const MyApp());
});
}
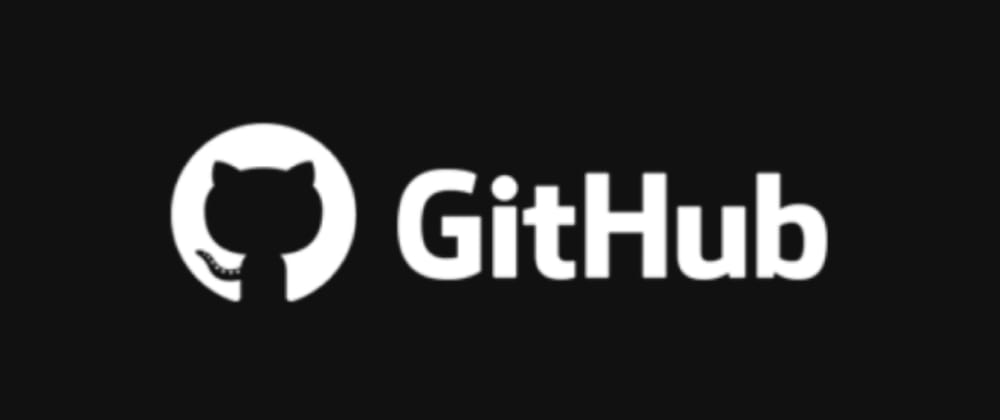