Calculating the size dynamically
To calculate the height or the width of a widget dynamically instead of using fixed values, we need to
know
first how
much size is available.
This is where MediaQuery comes into action, we can get information about the
current
device size, as well as
user preferences, and design your layout accordingly. MediaQuery provides a higher-level view of the
current
app’s screen size and can also give more detailed information about the device and its layout
preferences.
We have to connect it to our context ( the metadata object with some information about our widget and
its
position in the tree ).
Example :
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
child: const Text(
"50% <-->",
),
width: MediaQuery.of(context).size.width * 0.5,
color: Colors.redAccent,
),
Container(
child: const Text(
"70% <-->",
),
width: MediaQuery.of(context).size.width * 0.7,
color: Colors.orangeAccent,
),
SizedBox(height: MediaQuery.of(context).size.height * 0.4),
Container(
child: const Text(
"30% ↑ 90% <-->",
),
height: MediaQuery.of(context).size.height * 0.3,
width: MediaQuery.of(context).size.width * 0.9,
color: Colors.pink,
),
],
)
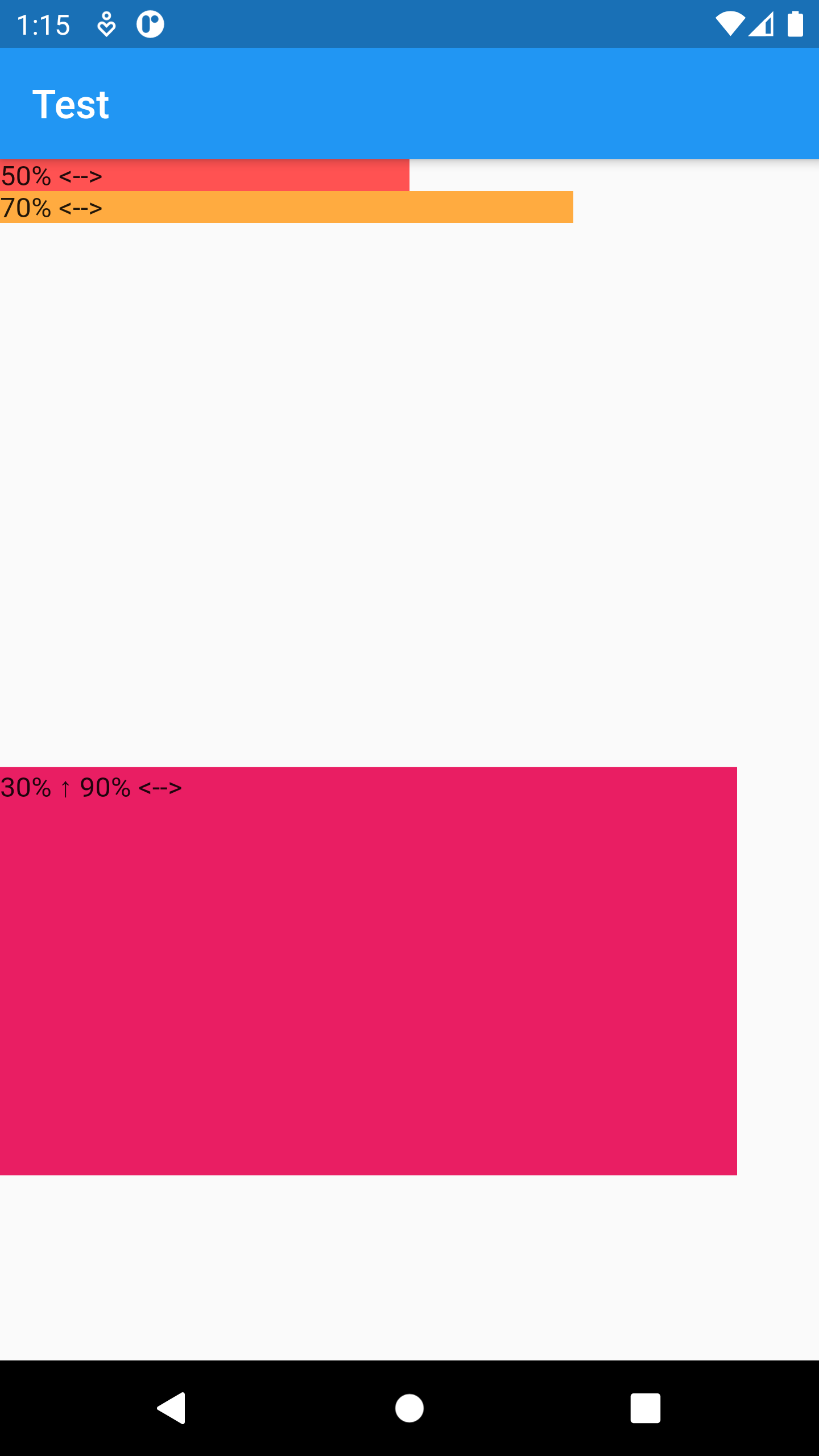
Exercise :
Try to do this using Image.network, MediaQuery and IconButton.
Solution :
class _MyHomePageState extends State<MyHomePage> {
var size, height, width, dog = 0.5, cat = 0.5;
@override
Widget build(BuildContext context) {
size = MediaQuery.of(context).size;
height = size.height;
width = size.width;
return Scaffold(
appBar: AppBar(
title: const Text("Test"),
),
body: Center(
child: Column(
children: [
Image.network(
"https://www.sciencemag.org/sites/default/files/styles/article_main_large/public/dogs_1280p_0.jpg?itok=5P-MAjr-",
width: width * dog,
),
dog == 1
? IconButton(
onPressed: () {
setState(() {
dog = 0.5;
});
},
icon: const Icon(Icons.zoom_out))
: IconButton(
onPressed: () {
setState(() {
dog = 1;
});
},
icon: const Icon(Icons.zoom_in)),
Image.network(
"https://static.scientificamerican.com/sciam/cache/file/92E141F8-36E4-4331-BB2EE42AC8674DD3_source.jpg",
width: width * cat,
),
cat == 1
? IconButton(
onPressed: () {
setState(() {
cat = 0.5;
});
},
icon: const Icon(Icons.zoom_out))
: IconButton(
onPressed: () {
setState(() {
cat = 1;
});
},
icon: const Icon(Icons.zoom_in)),
],
),
),
);
}
}