Adding Shared Preferences To App4
- Installing
- Import the package in the main
- Create a key
- Saving The Best Score
- Getting The Best Score
- Test the app
- Code Source
Run this command:
flutter pub add shared_preferences
This will add a line like this to your package's pubspec.yaml (and run an implicit flutter pub get):
dependencies:
shared_preferences: ^2.0.9
Or visit shared_preferences - pub.dev
Now run pub get from the command line:
flutter pub get
You’re now ready to store data.
import 'package:shared_preferences/shared_preferences.dart';
All preferences need to use a unique key or they’ll be overwritten. Here, you’re simply defining a constant for the preference key.
static const String bestScorekey = 'bestScore';
To understand the code you’ll add next, you need to know a bit about running code in
the background.
Most modern UIs have a main thread that runs the UI code. Any code that takes a
long time needs to run on a different thread or process so it doesn’t block the UI.
Dart uses a technique similar to JavaScript to achieve this. The language includes
these two keywords:
• async
• await
Check these lessons :
void saveNewBestScore(int value) async {
//1
final prefs = await SharedPreferences.getInstance();
//2
prefs.setInt(bestScorekey, value);
}
Here, you use the async keyword to indicate that this method will run
asynchronously. It also:
1. Uses the await keyword to wait for an instance of SharedPreferences.
2. Saves the best score using the prefSearchKey key.
Next, call it in the check answer method:
void checkAnswer() {
if (answer == correctCountry) {
setState(() {
score++;
prepareCountries();
});
} else {
if (score > bestScore) {
bestScore = score;
///add this here
saveNewBestScore(score);
}
setState(() {
playing = false;
});
prepareCountries();
}
}
void getBestScore() async {
//1
final prefs = await SharedPreferences.getInstance();
//2
if (prefs.containsKey(bestScorekey)) {
//3
bestScore = prefs.getInt(bestScorekey);
}
}
This method is also asynchronous. Here, you:
1. Use the await keyword to wait for an instance of SharedPreferences.
2. Check if a preference for the best score already exists.
3. Get the best score.
Finally, right after super.initState() in initState() call it:
@override
void initState() {
super.initState();
prepareCountries();
getBestScore();
}
This loads the previous best score when the user restarts the app.
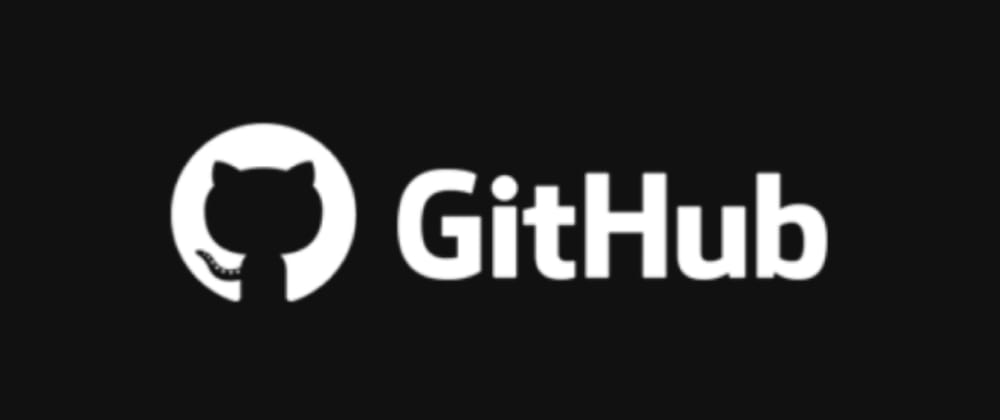