Content depending on the available device size
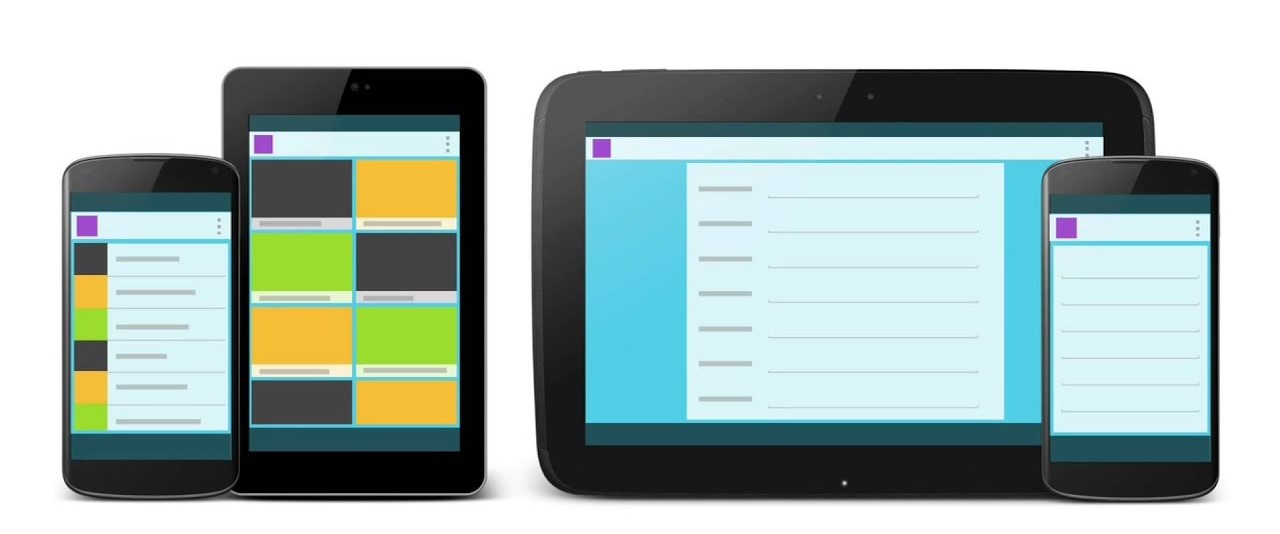
A multitude of different screen sizes exist across phones, tablets, desktops, TVs, and even wearables. Screen sizes are always changing, so it's important that your app can adapt to any screen size, today or in the future. In addition, devices have different features with which we interact with them. For example some of your visitors will be using a touchscreen others will be using keyboard and mouse. Modern responsive design considers all of these things to optimize the experience for everyone
Exercise :
Try to make something like that where :
UCL Clubs 2019-20 file : Download
-
Width < 500 :
-
500 < Width < 700
-
Width > 700 with GridView crossAxisCount: width ~/ 300,
Solution :
import 'package:flutter/material.dart';
import './cl2019.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: "UCL Clubs 2019-20",
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
var width = MediaQuery.of(context).size.width;
return Scaffold(
appBar: AppBar(
title: const Text("UCL Clubs 2019-20"),
backgroundColor: const Color.fromRGBO(60, 60, 230, 1),
),
body: width > 700
? GridView.count(
crossAxisCount: width ~/ 300,
children: List.generate(uclClubs2019_20.length, (index) {
return Card(
margin: const EdgeInsets.all(8),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(25),
color:
Colors.accents[index % Colors.accents.length]),
width: double.maxFinite,
padding: EdgeInsets.all(width * 0.01),
child: Text(
uclClubs2019_20[index]['code'].toString(),
textAlign: TextAlign.center,
style: const TextStyle(
color: Colors.white, fontSize: 35),
),
),
const Divider(),
Text(
uclClubs2019_20[index]['name'].toString(),
style: const TextStyle(
fontSize: 25, fontWeight: FontWeight.bold),
),
const Divider(),
Text(
uclClubs2019_20[index]['country'].toString(),
style: const TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.lightBlue),
),
const Divider(),
GestureDetector(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Favorite",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color:
uclClubs2019_20[index]['favorite'] == true
? Colors.pink
: Colors.grey),
),
uclClubs2019_20[index]['favorite'] == true
? const Icon(
Icons.favorite,
color: Colors.pink,
)
: const Icon(
Icons.favorite_border_outlined,
color: Colors.grey,
)
],
),
onTap: () {
setState(() {
uclClubs2019_20[index]['favorite'] =
uclClubs2019_20[index]['favorite'] == true
? false
: true;
});
},
)
],
),
);
}),
)
: ListView.builder(
itemCount: uclClubs2019_20.length,
itemBuilder: (ctx, index) {
return Card(
child: ListTile(
leading: Container(
padding: const EdgeInsets.all(8),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),
color: Colors.accents[index % Colors.accents.length]),
child: Text(
uclClubs2019_20[index]['code'].toString(),
textAlign: TextAlign.center,
style:
const TextStyle(color: Colors.white, fontSize: 25),
),
),
title: Text(
uclClubs2019_20[index]['name'].toString(),
style: const TextStyle(
fontSize: 20, fontWeight: FontWeight.bold),
),
subtitle: Text(
uclClubs2019_20[index]['country'].toString(),
style: const TextStyle(
fontSize: 15,
fontWeight: FontWeight.bold,
color: Colors.lightBlue),
),
trailing: GestureDetector(
child: FittedBox(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
width > 500
? Text(
"Favorite",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: uclClubs2019_20[index]
['favorite'] ==
true
? Colors.pink
: Colors.grey),
)
: Container(),
uclClubs2019_20[index]['favorite'] == true
? const Icon(
Icons.favorite,
color: Colors.pink,
)
: const Icon(
Icons.favorite_border_outlined,
color: Colors.grey,
)
],
),
),
onTap: () {
setState(() {
uclClubs2019_20[index]['favorite'] =
uclClubs2019_20[index]['favorite'] == true
? false
: true;
});
},
),
),
);
},
),
);
}
}