Inheritance
- What is Inheritance?
Single Inheritance
Multilevel Inheritance
Hierarchical Inheritance
- Syntax
- Single Level Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
Inheritance is a mechanism in which one class acquires the property of another class.
For example, a child inherits the traits of his/her parents.
With inheritance, we can reuse the variables and methods of the existing class.
Hence, inheritance facilitates Reusability and is an important concept of OOPs.
Child Class : The class that extends the features of another class is known as child class, sub class or derived class.
Parent Class : The class whose properties and functionalities are used(inherited) by another class is known as parent class, super class or Base class.
The inheritance can be mainly three types:
Dart doesn’t support Multiple Inheritance
//body of child class
}
A single subclass extends from a single superclass.
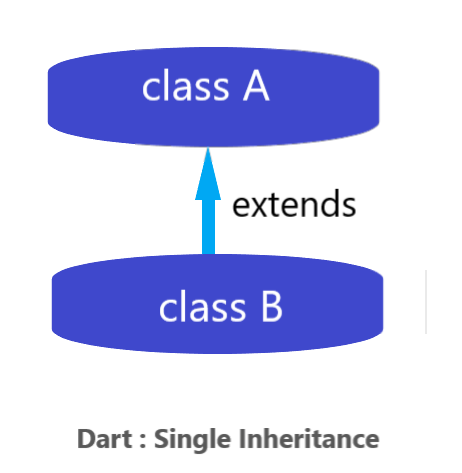
Example:
class Person {
String name;
int id;
void printName() {
print(name);
}
}
class Student extends Person {
int nb;
void printId() {
print(id);
}
void setName(String x) {
name = x;
}
}
void main() {
var student1 = Student();
student1.setName("Sam");
student1.printName();
student1.id = 1234;
student1.printId();
student1.nb = 7;
}
1234
A subclass extends from a superclass and then the same subclass acts as a superclass for another class.
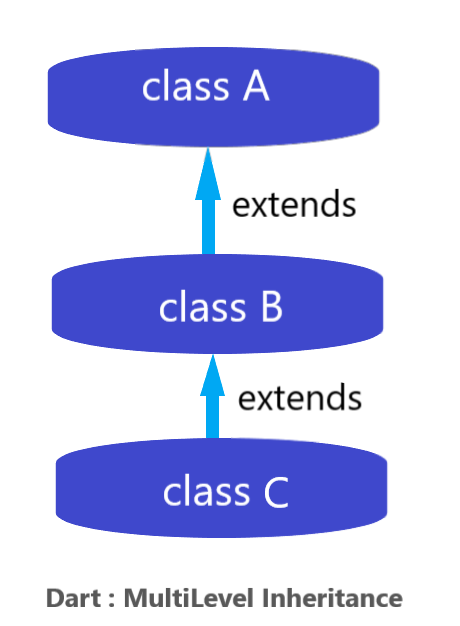
Example:
class Person {
String name;
int id;
void printName() {
print(name);
}
}
class Student extends Person {
int nb;
void printId() {
print(id);
}
void setName(String x) {
name = x;
}
}
class ClubMember extends Student {
String role;
void setRole(String x) {
role = x;
}
}
void main() {
var member1 = ClubMember();
member1.setName("Sam");
member1.printName();
member1.id = 1234;
member1.printId();
member1.nb = 7;
member1.setRole("Admin");
print(member1.role);
}
1234
Admin
Multiple subclasses extend from a single superclass.
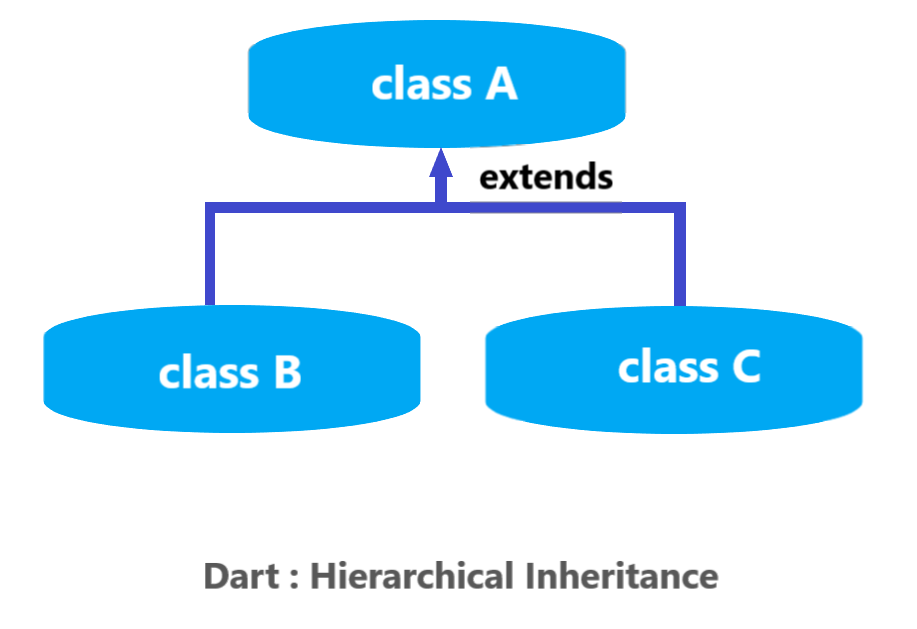
Example:
class Person {
String name;
int id;
void printName() {
print(name);
}
}
class Student extends Person {
int nb;
void printId() {
print(id);
}
void setName(String x) {
name = x;
}
}
class ClubMember extends Person {
String role;
void setRole(String x) {
role = x;
}
}
void main() {
var member1 = ClubMember();
member1.name = "Adem";
member1.printName();
member1.setRole("Founder");
print(member1.role);
var student1 = Student();
student1.setName("Elon");
student1.printName();
student1.nb = 345;
print(student1.nb);
}
Founder
Elon
345