Lists
- Definition
- Syntax
- Example
- Types
- Syntax
- Example
- Syntax
- Example
- Inserting Elements
- Updating Elements
- Removing Elements
- Other Methods
- Properties
- Methods
Dart List is similar to an array, which is an ordred group of objects. The list is used to hold multiple values in single variable.
var list_name = [value1,value2,value3,...,valueN];
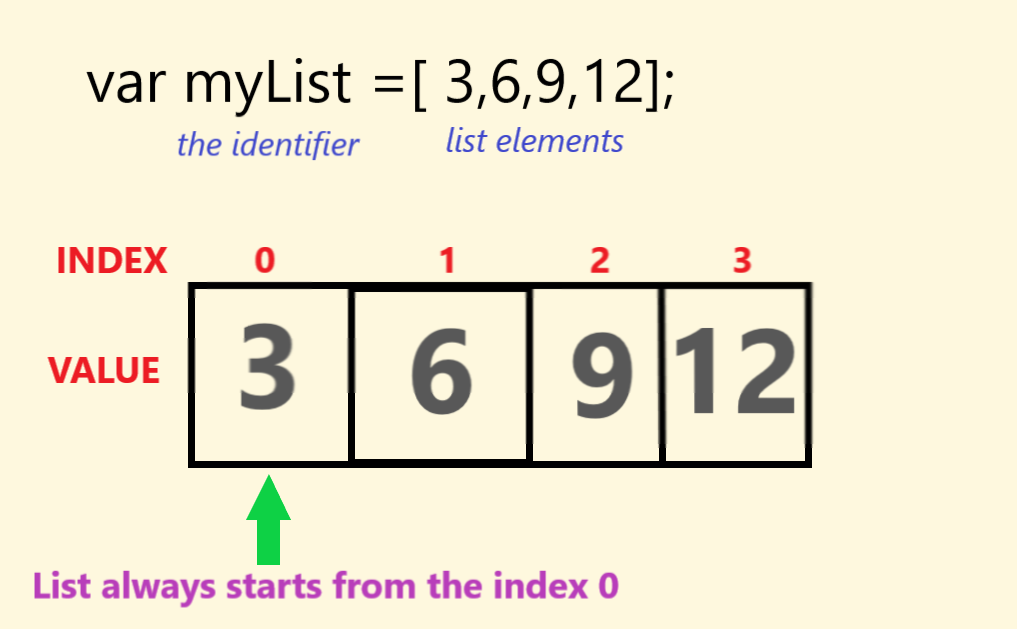
The Dart list can be categorized into two types :Fixed Length List & Growable List.
I) Fixed Length List
The fixed length lists have fixed length. We cannot change the size at runtime.
var list_name= new List(size); // declaring
list_name[index]=value; //Initializing
void main() {
var lst = new List (4);
lst[0] = 3;
lst[1] = 6;
lst[2] = 9;
lst[3] = 12;
print(lst[2]);
print(lst);
}
[3, 6, 9, 12]
II) Growable List
The list is declared without giving fixed size. The size of the Growable list can be modified at the runtime.
var list_name= new List(); // declaring an empty list (size=0)
//Initializing
var list_name=[value1, value2,..,valueN];
list_name[index]=value;
void main( ) {
var lst = [5, 10, 15, 20];
print( "the list before : $lst ");
lst.add ( 25);
print( "the list after : $lst ");
}
the list after : [5, 10, 15, 20, 25]
add(value)
Adds value to the end of this list, extending the length by one.
addAll(values)
Appends multiple elements to the end of this list.
insert(index,element)
Inserts the element at poition index in this list.
insertAll(index,values)
Inserts multiple elements at position index in this list.
void main() {
var list = [1, 4, 7];
print(list);
list.add(9);
print(list);
list.addAll([13, 17, 19]);
print(list);
list.insert(2, 5);
print(list);
list.insertAll(6, [14, 15, 16]);
print(list);
}
[1, 4, 7]
[1, 4, 7, 9]
[1, 4, 7, 9, 13, 17, 19]
[1, 4, 5, 7, 9, 13, 17, 19]
[1, 4, 5, 7, 9, 13, 14, 15, 16, 17, 19]
list[index]=newValue
Change the value by accessing to the element with its
replaceRange(start,end,values)
Removes the objects in the range start inclusive to end exclusive and inserts the contents of replacement in its place.
void main( ) {
var list = [1, 4, 5, 7, 9, 13, 17, 19];
print(list);
list[ 1] = 2;
print(list);
list.replaceRange (2, 8, [3, 4, 5, 6]);
print(list);
}
[1, 2, 5, 7, 9, 13, 17, 19]
[1, 2, 3, 4, 5, 6]
remove(value)
Removes the first occurrence of value from this list.
removeAt(index)
Removes the object at position index from this list.
removeRange(start,end)
Removes the objects in the range start inclusive to end exclusive.
clear()
Removes all objects from this list; the length of the list becomes zero.
void main( ) {
var list = [1, 2, 3, 4, 5, 6];
print(list);
list.remove (4);
print(list);
list.remove (9);
print(list);
list.removeAt (1);
print(list);
list.removeRange (2, 4);
print(list);
list.clear ();
print(list);
}
[1, 2, 3, 5, 6]
[1, 2, 3, 5, 6]
[1, 3, 5, 6]
[1, 3]
[]
contains(element)
Returns true if the collection contains an element equal to element.
indexOf(element)
Returns the first index of element in this list.
shuffle()
Shuffles the elements of this list randomly.
sublist(start,[end])
Returns a new list containing the elements between start and end.
sublist(start): Returns a new list containing the elements from start to the end of the list.
void main() {
var list = [1, 3, 5, 7, 9];
print(list);
print(list.contains(3));
print(list.contains(8));
print(list.indexOf(5));
print(list.indexOf(15));
list.shuffle();
print(list);
list.shuffle();
print(list);
var list2 = list.sublist(2, 4);
print(list2);
}
[1, 3, 5, 7, 9]
true
false
2
-1
[7, 5, 9, 3, 1]
[9, 1, 7, 3, 5]
[7, 3]
The next parts are not important to know to start using lists. You may need one of them later.
Property | Description |
---|---|
first | Returns the first element. |
hashCode | The hash code for this object. |
isEmpty | Returns true if there are no elements in this collection. |
isNotEmpty | Returns true if there is at least one element in this collection. |
iterator | Returns a new Iterator that allows iterating the elements of this Iterable. |
last | Returns the last element. |
length | The number of objects in this list. |
reversed | Returns an Iterable of the objects in this list in reverse order. |
runtimeType | A representation of the runtime type of the object. |
single | Checks that this iterable has only one element, and returns that element. |
Method | Description |
---|---|
add(value) | Adds value to the end of this list, extending the length by one. |
addAll(values) | Appends all objects of iterable to the end of this list. |
any | Checks whether any element of this iterable satisfies test. |
asMap() | Returns an unmodifiable Map view of this. |
cast |
Returns a view of this list as a list of R instances. |
clear() | Removes all objects from this list; the length of the list becomes zero. |
contains(element) | Returns true if the collection contains an element equal to element. |
elementAt(index) | Returns the indexth element. |
every(bool test(E element)) | Checks whether every element of this iterable satisfies test. |
expand |
Expands each element of this Iterable into zero or more elements. |
fillRange(int start, int end, [E? fillValue]) | Sets the objects in the range start inclusive to end exclusive to the given fillValue. |
firstWhere(bool test(E element), {E orElse()}) | Returns the first element that satisfies the given predicate test. |
fold |
Reduces a collection to a single value by iteratively combining each element of the collection with an existing value |
followedBy(Iterable |
Returns the lazy concatentation of this iterable and other. |
forEach(void f(E element)) | Applies the function f to each element of this collection in iteration order. |
getRange(int start, int end) | Returns an Iterable that iterates over the objects in the range start inclusive to end exclusive. |
indexOf(E element, [int start = 0]) | Returns the first index of element in this list. |
indexWhere(bool test(E element), [int start = 0]) | Returns the first index in the list that satisfies the provided test. |
insert(int index, E element) | Inserts the object at position index in this list. |
insertAll(int index, Iterable |
Inserts all objects of iterable at position index in this list. |
join([String separator = ""]) | Converts each element to a String and concatenates the strings. |
lastIndexOf(E element, [int? start]) | Returns the last index of element in this list. |
lastIndexWhere(bool test(E element), [int? start]) | Returns the last index in the list that satisfies the provided test. |
lastWhere(bool test(E element), {E orElse()}) | Returns the last element that satisfies the given predicate test. |
map |
Returns a new lazy Iterable with elements that are created by calling f on each element of this Iterable in iteration order. |
noSuchMethod(Invocation invocation) | Invoked when a non-existent method or property is accessed. |
reduce(E combine(E value, E element)) | Reduces a collection to a single value by iteratively combining elements of the collection using the provided function. |
remove(Object? value) | Removes the first occurrence of value from this list. |
removeAt(int index) | Removes the object at position index from this list. |
removeLast() | Pops and returns the last object in this list. |
removeRange(int start, int end) | Removes the objects in the range start inclusive to end exclusive. |
removeWhere(bool test(E element)) | Removes all objects from this list that satisfy test. |
replaceRange(int start, int end, Iterable |
Removes the objects in the range start inclusive to end exclusive and inserts the contents of replacement in its place. |
retainWhere(bool test(E element)) | Removes all objects from this list that fail to satisfy test. |
setAll(int index, Iterable |
Overwrites objects of this with the objects of iterable, starting at position index in this list. |
setRange(int start, int end, Iterable |
Copies the objects of iterable, skipping skipCount objects first, into the range start, inclusive, to end, exclusive, of the list. |
shuffle([Random? random]) | Shuffles the elements of this list randomly. |
singleWhere(bool test(E element), {E orElse()}) | Returns the single element that satisfies test. |
skip(int count) | Returns an Iterable that provides all but the first count elements. |
skipWhile(bool test(E value)) | Returns an Iterable that skips leading elements while test is satisfied. |
sort([int compare(E a, E b)]) | Sorts this list according to the order specified by the compare function. |
sublist(int start, [int? end]) | Returns a new list containing the elements between start and end. |
take(int count) | Returns a lazy iterable of the count first elements of this iterable. |
takeWhile(bool test(E value)) | Returns a lazy iterable of the leading elements satisfying test. |
toList({bool growable: true}) | Creates a List containing the elements of this Iterable. |
toSet() | Creates a Set containing the same elements as this iterable. |
toString() | Returns a string representation of this object. |
where(bool test(E element)) | Returns a new lazy Iterable with all elements that satisfy the predicate test. |
whereType |
Returns a new lazy Iterable with all elements that have type T. |