Exercise
Create the following directory tree :

- msg.dart
- trigo.dart
- cal.dart
- main.dart
Create two fuctions the first print "Program starts" and the second print "Program ends".
Create three functions that print the sin , cos and tan of a given number using math library.
Create two functions that print the square and the logarithm of a given number using math library.
- Import msg.dart and cal.dart.
- Import trigo.dart with a perfix "trigo".
- Use all functions inside all these 3 files.
Solution:
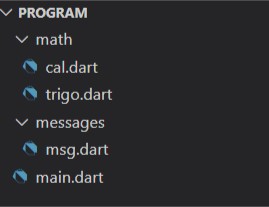
- msg.dart
- trigo.dart
- cal.dart
- main.dart
void printStart() {
print("Program start");
}
void printEnd() {
print("Program end");
}
import 'dart:math';
void printSin(double x) {
print("sin($x) = ${sin(x)}");
}
void printCos(double x) {
print("cos($x) = ${cos(x)}");
}
void printTan(double x) {
print("Tan($x) = ${tan(x)}");
}
import 'dart:math';
void printSqrt(double x) {
print("the sqrt of $x is ${sqrt(x)}");
}
void printLog(double x) {
print("the log of $x is ${log(x)}");
}
import './messages/msg.dart';
import './math/cal.dart';
import './math/trigo.dart' as trigo;
void main() {
printStart();
trigo.printSin(0.5);
trigo.printCos(0.75);
trigo.printTan(0.25);
printSqrt(9);
printLog(1);
printEnd();
}
sin(0.5) = 0.479425538604203
cos(0.75) = 0.7316888688738209
Tan(0.25) = 0.25534192122103627
the sqrt of 9.0 is 3.0
the log of 1.0 is 0.0
Program end